Does your internet feel slower in the evenings or on weekends? This Python script downloads a 3MB file every 5 minutes to log your download speed in a CSV file. You can change the frequency or choose a different file by editing the script.
### Internet speed test script for Python 2.6+ ### ### This runs continuously downloading the test file every 5 minutes to generate ### a csv file that you can use to generate a scatter chart in Excel. ### from __future__ import print_function from time import time, sleep from datetime import datetime from os.path import exists, expanduser, join try: from urllib.request import urlopen # Python 3 except ImportError: from urllib2 import urlopen # Python 2 output_file = join(expanduser("~"),"internet_speed.csv") ### CONFIGURATION repeat_minutes = 5 download_file = "http://home.exetel.com.au/speedtest/storm_3mb.jpg" file_bytes = 3062059 # This must be the exact size of the download file in bytes if you want accurate results! ### if not exists(output_file): try: with open(output_file, "w") as f: f.write("Time,Speed (MB/s)\n") except IOError: print ("Please edit the configuration. Output file location does not exist.") input() exit() while True: start_time = time() urlopen(download_file).read() seconds_taken = time() - start_time mbytes_per_second = str( round(file_bytes / seconds_taken / 1024 / 1024, 2) ) print (mbytes_per_second," MB/s") with open(output_file, "a") as f: f.write(datetime.now().strftime("%Y-%m-%d %H:%M:00")+','+mbytes_per_second+'\n') print ("Waiting ",repeat_minutes," minutes.") sleep((repeat_minutes * 60) - (time() - start_time))
Download speedtest.py
You will find the output file in C:\users\username on Windows, or in /home/username on Linux. It should look like this:
Time,Speed (MB/s)
2014-11-03 23:00:22,0.161
2014-11-03 23:05:36,0.96.5
2014-11-03 23:10:17,0.217
2014-11-03 23:15:23,0.149
2014-11-03 23:20:29,0.152
Import the file in Excel or Gnumeric and make a chart!
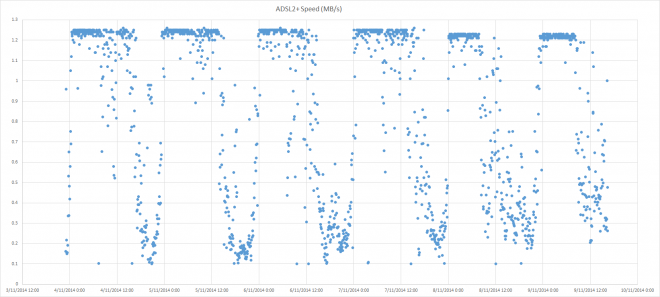
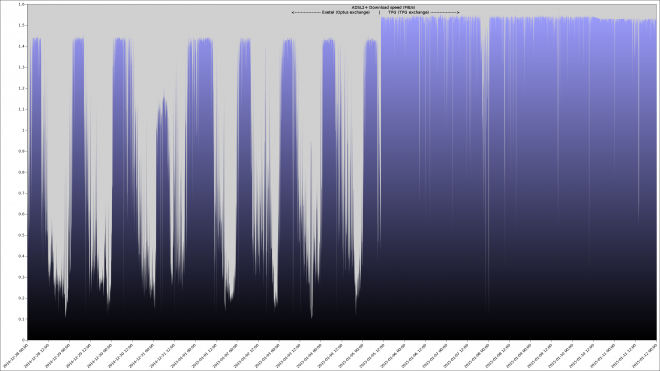